Handling time zones in E2E tests
You may encounter unexpected errors if you have been developing and running test code locally and then start using a runner in a different time zone. Here are a few things to try to address these issues.
- Ensure tests are time-independent. Most modern code languages have time/date libraries to convert between time zones quickly. It is advisable to standardize the time retrieved from both the application's interface and the runner's system by converting it to a chosen time zone. This way, you can make your actions and assertions on a common, expected time.
from datetime import datetime
import pytz
# Get the system time in Pacific
system_pacific_now = datetime.now(pytz.timezone('America/Los_Angeles'))
# Set/Get the UI times (expected Pacific)
ui_date_format = "%Y-%m-%d %H:%M:%S"
appointment_time = system_pacific_now + timedelta(days=1)
... <set time for appointment>
expected_time = driver.find_element(By.ID, 'scheduledTime').text
# returns "2025-04-02 10:30:00"
returned_appointment_time = datetime.strptime(expected_time, ui_date_format)
# Format the times as a common string
common_format = "%Y-%m-%d %H:%M"
actual_time = appointment_time.strftime(common_format)
assert returned_appointment_time.strftime(common_format) == actual_time
- Modify the browser’s time zone. If your application is limited to operations in certain time zones, changing the browser’s time zone on the test runner may yield beneficial results. To achieve this, you can use browser options when initializing the driver.
driver = webdriver.Chrome()
tz_params = {'timezoneId': 'America/Los_Angeles'}
driver.execute_cdp_cmd('Emulation.setTimezoneOverride', tz_params)
Example for Python and Selenium
// playwright.config.ts
import { defineConfig } from '@playwright/test';
export default defineConfig({
// ... other configurations
use: {
timezoneId: 'America/Los_Angeles',
},
});
Example for Playwright
// cypress/support/e2e.js
Cypress.on('test:before:run', () => {
Cypress.automation('remote:debugger:protocol', {
command: 'Emulation.setTimezoneOverride',
params: {
timezoneId: 'America/Los_Angeles',
},
});
});
Example for Cypress
For Webdriverio, it is a little more complex. First, make sure you have puppeteer-core added npm install puppeteer-core
. Then, make a call to apply the changes to the desired page or all pages.
💡
If using Bidi instead of the classic driver and spawning the Bidi tab, the page order may be random so I would recommend to add additional code to guarantee the page you want.
// features/step-definitions/steps.ts
BeforeAll(async () => {
const puppeteerBrowser = await browser.getPuppeteer()
await browser.call(async () => {
const page = (await puppeteerBrowser.pages())[0];
page.emulateTimezone('America/Los_Angeles');
})
})
Example for WDIO
To verify the browser time change, write a simple test that goes to a site like https://time.gov and checks if the local clock matches the desired time zone.
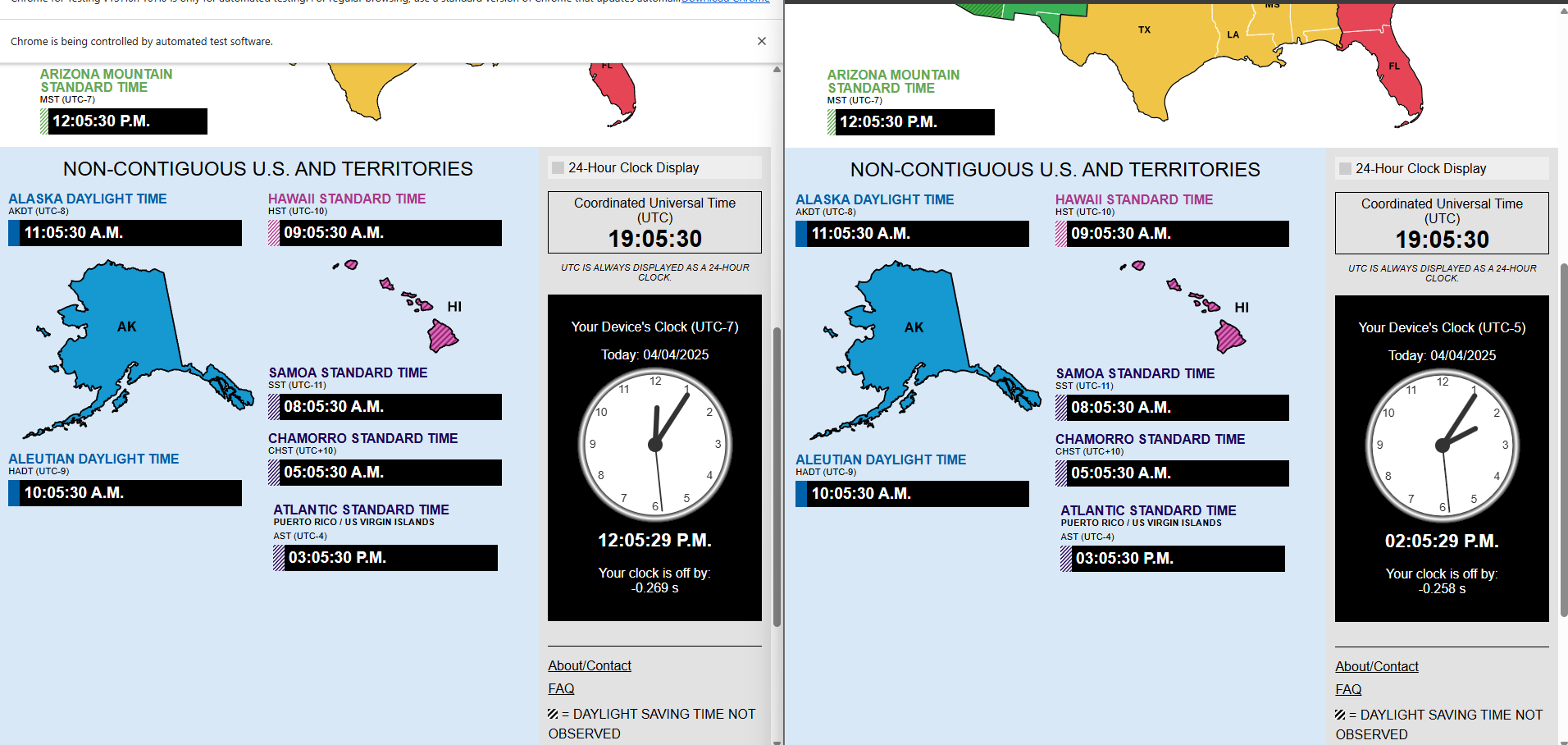